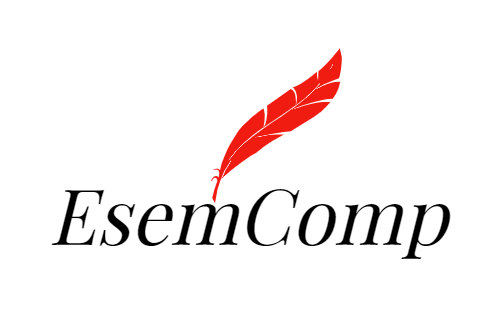
ESEM EFA
esem_efa.Rd
Wrapper around psych::fa() for running ESEM-like exploratory factor analysis.
Usage
esem_efa(
data,
nfactors,
target = "none",
bifactor = FALSE,
fm = "pa",
targetAlgorithm = "targetQ",
...
)
Arguments
- data
Raw data.frame or matrix. Subjects x Items data or item covariance matrix.
- nfactors
An integer. Number of factors to extract (including G when bifactor).
- target
Target rotation matrix. Usually obtained with make_target(). Defaults to "none", in which case geominQ rotation is used.
- bifactor
Logical. Set to TRUE if model is bifactor.
- fm
Factor extraction method. Defaults to "Principal Axis", see psych::fa() for alternatives.
- targetAlgorithm
Character vector. Factor rotation to use with target matrix. Defaults to oblique target rotation ("targetQ"). An alternative is "TargetT", for orthogonal target rotation. Automatically set to orthogonal target rotation if
bifactor = TRUE
.- ...
Additional parameters passed to psych::fa().
Details
Facilitates ESEM-like exploratory factor analyses.
In the simplest case, a (oblique) Geomin rotation is used after factor extraction.
The user can control the value of Geomin delta
(aka epsilon
in the literature)
by specifying the desired number (usually between 0.1 and 0.9) with delta
in ...
(see examples).
To run a factor extraction with target rotation, a target rotation matrix (items x factors)
must be supplied to target
. Matrix cells should show zeros where loadings are expected
to be as close to zero as possible and NA
where loadings should be freely estimated.
One can create such a matrix by hand with base::matrix(), but check the helping
function make_target() included with this package. The default target rotation
is oblique, but can be changed to the orthogonal alternative
by setting targetAlgorithm = "TargetT"
.
To run a factor extraction bifactor target rotation, a bifactor target rotation matrix must be
supplied to target
and bifactor
should be set to TRUE
.
Examples
#use Tucker 9 cognitive variables cov matrix
tucker <- psych::Tucker
# esem with Geomin rotation
esem_efa(tucker, 2)
#> Loading required namespace: GPArotation
#> Factor Analysis using method = pa
#> Call: psych::fa(r = data, nfactors = nfactors, rotate = "geominQ",
#> fm = fm)
#> Standardized loadings (pattern matrix) based upon correlation matrix
#> PA1 PA2 h2 u2 com
#> t42 -0.03 0.70 0.48 0.52 1.0
#> t54 0.02 0.71 0.51 0.49 1.0
#> t45 0.91 -0.03 0.81 0.19 1.0
#> t46 0.86 -0.06 0.71 0.29 1.0
#> t23 -0.01 0.71 0.50 0.50 1.0
#> t24 0.00 0.74 0.55 0.45 1.0
#> t27 0.12 0.59 0.42 0.58 1.1
#> t10 0.76 0.13 0.68 0.32 1.1
#> t51 0.78 0.06 0.65 0.35 1.0
#>
#> PA1 PA2
#> SS loadings 2.84 2.47
#> Proportion Var 0.32 0.27
#> Cumulative Var 0.32 0.59
#> Proportion Explained 0.53 0.47
#> Cumulative Proportion 0.53 1.00
#>
#> With factor correlations of
#> PA1 PA2
#> PA1 1.00 0.42
#> PA2 0.42 1.00
#>
#> Mean item complexity = 1
#> Test of the hypothesis that 2 factors are sufficient.
#>
#> The degrees of freedom for the null model are 36 and the objective function was 4.49
#> The degrees of freedom for the model are 19 and the objective function was 0.07
#>
#> The root mean square of the residuals (RMSR) is 0.02
#> The df corrected root mean square of the residuals is 0.03
#>
#> Fit based upon off diagonal values = 1
#> Measures of factor score adequacy
#> PA1 PA2
#> Correlation of (regression) scores with factors 0.96 0.91
#> Multiple R square of scores with factors 0.92 0.83
#> Minimum correlation of possible factor scores 0.83 0.67
# esem with Geomin rotation setting the rotation delta (aka epsilon)
esem_efa(tucker, 2, delta = .5)
#> Factor Analysis using method = pa
#> Call: psych::fa(r = data, nfactors = nfactors, rotate = "geominQ",
#> fm = fm, delta = 0.5)
#> Standardized loadings (pattern matrix) based upon correlation matrix
#> PA1 PA2 h2 u2 com
#> t42 0.03 0.68 0.48 0.52 1.0
#> t54 0.08 0.69 0.51 0.49 1.0
#> t45 0.89 0.03 0.81 0.19 1.0
#> t46 0.84 0.00 0.71 0.29 1.0
#> t23 0.05 0.69 0.50 0.50 1.0
#> t24 0.06 0.72 0.55 0.45 1.0
#> t27 0.16 0.58 0.42 0.58 1.2
#> t10 0.76 0.17 0.68 0.32 1.1
#> t51 0.77 0.10 0.65 0.35 1.0
#>
#> PA1 PA2
#> SS loadings 2.86 2.45
#> Proportion Var 0.32 0.27
#> Cumulative Var 0.32 0.59
#> Proportion Explained 0.54 0.46
#> Cumulative Proportion 0.54 1.00
#>
#> With factor correlations of
#> PA1 PA2
#> PA1 1.00 0.29
#> PA2 0.29 1.00
#>
#> Mean item complexity = 1
#> Test of the hypothesis that 2 factors are sufficient.
#>
#> The degrees of freedom for the null model are 36 and the objective function was 4.49
#> The degrees of freedom for the model are 19 and the objective function was 0.07
#>
#> The root mean square of the residuals (RMSR) is 0.02
#> The df corrected root mean square of the residuals is 0.03
#>
#> Fit based upon off diagonal values = 1
#> Measures of factor score adequacy
#> PA1 PA2
#> Correlation of (regression) scores with factors 0.95 0.91
#> Multiple R square of scores with factors 0.91 0.82
#> Minimum correlation of possible factor scores 0.82 0.65
# esem with oblique target rotation
target_mat <- make_target(9, list(f1 = c(1,2,5:7), f2 = c(3,4,8,9)))
esem_efa(tucker,2,target_mat)
#> Factor Analysis using method = pa
#> Call: psych::fa(r = data, nfactors = nfactors, rotate = targetAlgorithm,
#> fm = fm, Target = target)
#> Standardized loadings (pattern matrix) based upon correlation matrix
#> PA2 PA1 h2 u2 com
#> t42 -0.05 0.71 0.48 0.52 1.0
#> t54 0.01 0.71 0.51 0.49 1.0
#> t45 0.92 -0.05 0.81 0.19 1.0
#> t46 0.87 -0.08 0.71 0.29 1.0
#> t23 -0.03 0.72 0.50 0.50 1.0
#> t24 -0.01 0.74 0.55 0.45 1.0
#> t27 0.11 0.59 0.42 0.58 1.1
#> t10 0.76 0.12 0.68 0.32 1.0
#> t51 0.79 0.04 0.65 0.35 1.0
#>
#> PA2 PA1
#> SS loadings 2.85 2.46
#> Proportion Var 0.32 0.27
#> Cumulative Var 0.32 0.59
#> Proportion Explained 0.54 0.46
#> Cumulative Proportion 0.54 1.00
#>
#> With factor correlations of
#> PA2 PA1
#> PA2 1.00 0.45
#> PA1 0.45 1.00
#>
#> Mean item complexity = 1
#> Test of the hypothesis that 2 factors are sufficient.
#>
#> The degrees of freedom for the null model are 36 and the objective function was 4.49
#> The degrees of freedom for the model are 19 and the objective function was 0.07
#>
#> The root mean square of the residuals (RMSR) is 0.02
#> The df corrected root mean square of the residuals is 0.03
#>
#> Fit based upon off diagonal values = 1
#> Measures of factor score adequacy
#> PA2 PA1
#> Correlation of (regression) scores with factors 0.96 0.92
#> Multiple R square of scores with factors 0.92 0.84
#> Minimum correlation of possible factor scores 0.83 0.68
# esem with bifactor target rotation
bifactor_target_mat <- make_target(9, list(f1 = c(1,2,5:7), f2 = c(3,4,8,9)), TRUE)
esem_efa(tucker,3,bifactor_target_mat, maxit = 2000) #maxit needed for convergence
#> Factor Analysis using method = pa
#> Call: psych::fa(r = data, nfactors = nfactors, rotate = targetAlgorithm,
#> fm = fm, Target = target, maxit = 2000)
#> Standardized loadings (pattern matrix) based upon correlation matrix
#> PA3 PA2 PA1 h2 u2 com
#> t42 0.56 -0.02 0.44 0.51 0.49 1.9
#> t54 0.57 0.03 0.53 0.61 0.39 2.0
#> t45 0.36 0.83 0.03 0.82 0.18 1.4
#> t46 0.35 0.77 -0.07 0.71 0.29 1.4
#> t23 0.73 -0.06 0.13 0.55 0.45 1.1
#> t24 0.67 -0.01 0.29 0.53 0.47 1.4
#> t27 0.70 0.06 0.04 0.49 0.51 1.0
#> t10 0.48 0.67 -0.02 0.68 0.32 1.8
#> t51 0.38 0.71 0.06 0.66 0.34 1.5
#>
#> PA3 PA2 PA1
#> SS loadings 2.73 2.24 0.59
#> Proportion Var 0.30 0.25 0.07
#> Cumulative Var 0.30 0.55 0.62
#> Proportion Explained 0.49 0.40 0.11
#> Cumulative Proportion 0.49 0.89 1.00
#>
#> With factor correlations of
#> PA3 PA2 PA1
#> PA3 1 0.00 0.00
#> PA2 0 1.00 0.09
#> PA1 0 0.09 1.00
#>
#> Mean item complexity = 1.5
#> Test of the hypothesis that 3 factors are sufficient.
#>
#> The degrees of freedom for the null model are 36 and the objective function was 4.49
#> The degrees of freedom for the model are 12 and the objective function was 0.02
#>
#> The root mean square of the residuals (RMSR) is 0.01
#> The df corrected root mean square of the residuals is 0.01
#>
#> Fit based upon off diagonal values = 1
#> Measures of factor score adequacy
#> PA3 PA2 PA1
#> Correlation of (regression) scores with factors 0.87 0.92 0.64
#> Multiple R square of scores with factors 0.76 0.85 0.42
#> Minimum correlation of possible factor scores 0.53 0.70 -0.17